---세팅----
1. pom.xml > myBatis 주석해제
- 챕터 02. STS4 프로젝트 생성 에서 주석처리했던 pom.xml > myBatis 관련 의존성 주석을 해제
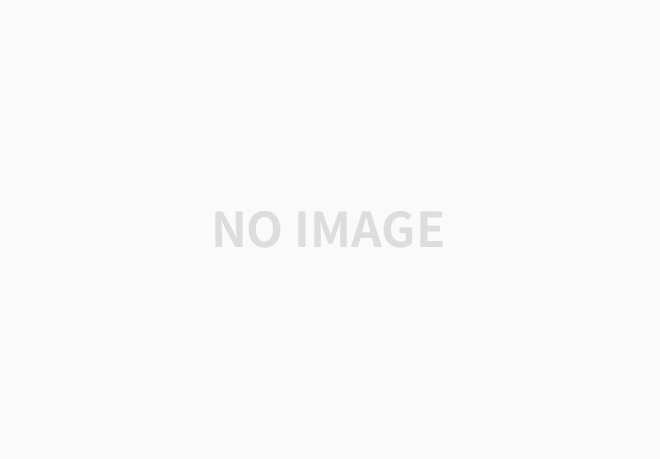
2. Mybatis-config.xml 생성
- src/main/resources 경로에 생성
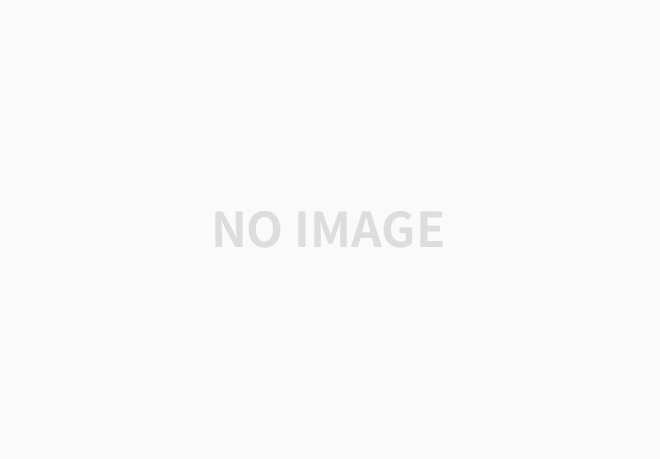
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<settings>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
</configuration>
3. 쿼리가 작성될 mapper 폴더 생성
- src./main/resources 경로에 추가
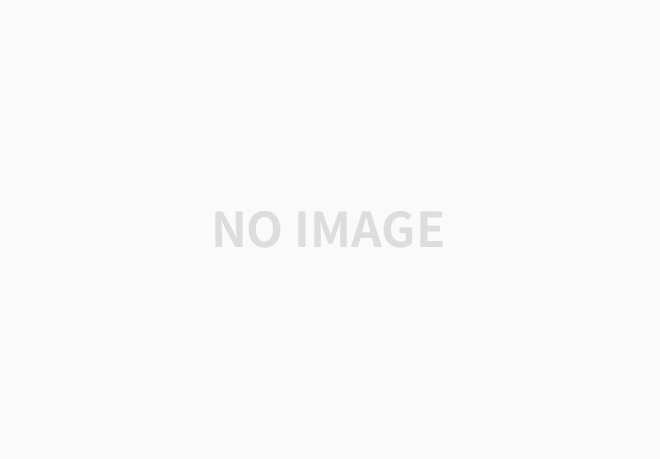
4.application.properties 추가
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/devboard?serverTimezone=UTC
spring.datasource.username=id
spring.datasource.password=password
mybatis.type-aliases-package=com.anse.web.model
mybatis.config-location=classpath:mybatis-config.xml
mybatis.mapper-locations=classpath:mapper/*.xml
---- 세팅 끝났으니 테스트 해보자--
1. sampleMapper.xml 생성
- src/main/resources/mapper 에 생성
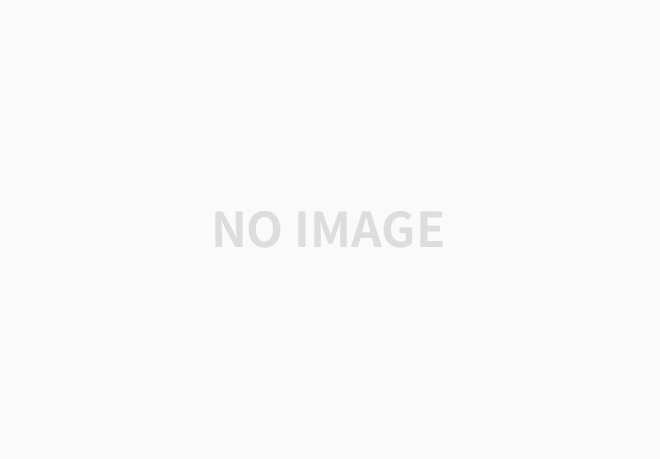
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.anse.web.mapper.SampleMapper">
<select id="getSample" resultType="SampleModel">
SELECT board_no
, title
, board_content
FROM t_board
</select>
</mapper>
2. 위 namespace 에 있는 경로와 SampleMapper 인터페이스 생성
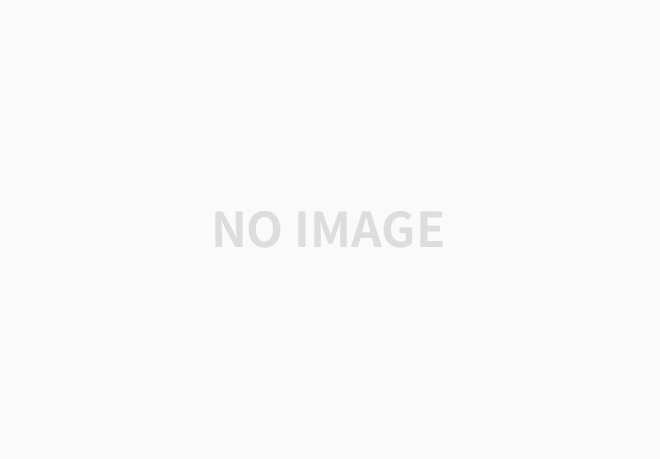
package com.anse.web.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
import org.springframework.stereotype.Repository;
import com.anse.web.model.SampleModel;
@Repository
@Mapper
public interface SampleMapper {
List<SampleModel> getSample();
}
3. src/main/java/service 패키지 생성 후 SampleService.java 생성
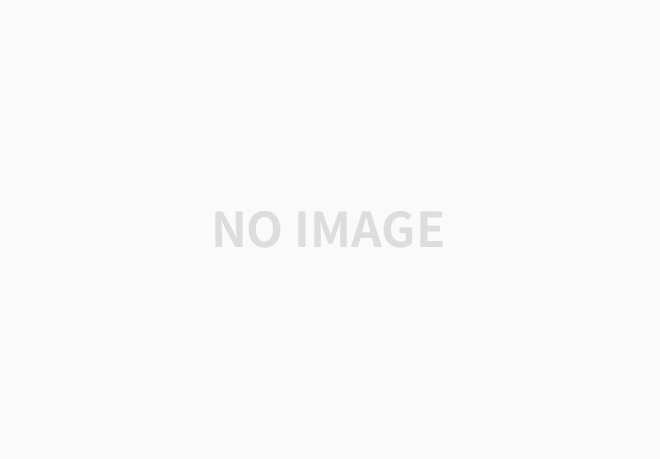
package com.anse.web.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.anse.web.mapper.SampleMapper;
import com.anse.web.model.SampleModel;
@Service
public class SampleService {
@Autowired
public SampleMapper sampleMapper;
public List<SampleModel> getSample() {
return sampleMapper.getSample();
}
}
4. SamplerController 수정
package com.anse.web.controller;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.servlet.ModelAndView;
import com.anse.web.model.SampleModel;
import com.anse.web.service.SampleService;
@Controller
public class SampleController {
@Autowired
SampleService sampleService;
@GetMapping("/sample")
public ModelAndView goHome(HttpServletRequest request, ModelAndView mv) {
mv.setViewName("sample/sample");
List<SampleModel> list = new ArrayList<>();
list = sampleService.getSample();
mv.addObject("list", list);
return mv;
}
}
5. 화면 확인
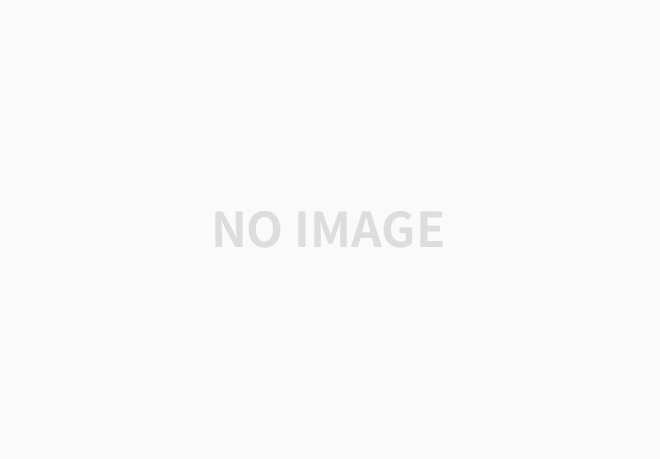
'MAC DEV > [mac]STS 웹 프로젝트 세팅' 카테고리의 다른 글
07. console 에 쿼리와 테이블 출력(log4jdbc) (0) | 2021.04.16 |
---|---|
05. mySql 설치 및 mySql 설정 (0) | 2021.04.14 |
04. Model 객체생성(builder 패턴) + 타임리프 적용 테스트 (0) | 2021.04.13 |
03. Thymeleaf(타임리프) 설정 및 기본 연동 (0) | 2021.04.12 |
02. STS4 프로젝트 생성 (Spring Starter Project) (0) | 2021.04.11 |